Dynamic Modals for Highlight
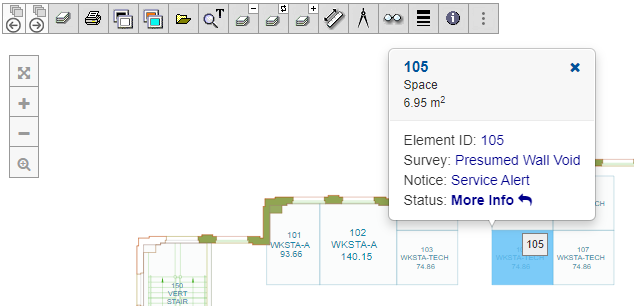
The section Custom Modals for Highlight describes in detail on how to set up user defined pop-up modals when interacting with an object space in the floor-plan for intelligent highlight. However, these modals are static in nature, they contains header information on the object clicked and the type of object, but the content of the modal itself keeps the original configuration.
CADViewer, also allows application programmers the ability to catch a call-back when an object is clicked, and dynamically set the content of the popup modal. This allows application programmers to interact with a database or back-end and serve object specific content back to the CADViewer canvas.
To define a call-back, initialize CADViewer with a call to the method cvjs_setCallbackForModalDisplay() to which two parameters myCustomPopUpBody and populateMyCustomPopUpBody in the call are methods that needs to be implemented separately in the code. (Refer to Custom Modals for Highlight to set up highlight color attributes, etc.)
var BaseAttributes = { /* keep as is */ };
var HighlightAttributes = { /* keep as is */ };
var SelectAttributes = { /* keep as is */ };
// Setting Space Object Modals Display to be based on a callback method -
cadviewer.cvjs_setCallbackForModalDisplay(true, myCustomPopUpBody, populateMyCustomPopUpBody);
my_cvjsPopUpBody = "";
cadviewer.cvjs_InitCADViewer_highLight_popUp_app("floorPlan", "/cadviewer/app/", BaseAttributes, HighlightAttributes, SelectAttributes, my_cvjsPopUpBody );
In this case the name of the call-back methods to populate the modal are:
- myCustomPopUpBody
- populateMyCustomPopUpBody
but the Application Programmers can of course use their own naming for these methods.
Typically, use the myCustomPopUpBody method as the placeholder for Modal content generation, and leave the method populateMyCustomPopUpBody, as population of this method is optional.
Custom method - myCustomPopUpBody
The method myCustomPopUpBody can contain any user defined content of the Space Modal at display. The method returns an object my_cvjsPopUpBody back to CADViewer, which is the HMTL code that is inserted into the Modal when the Modal is opened over the highlighted space object.
Typically the Application Programmen will use the rmid callback variable in myCustomPopUpBody that contains the Space ID of the clicked space, to make a call against a Database or content repository, and then create the content of the modal response accordingly.
Controls
To allow interaction with the content that has been passed over to myCustomPopUpBody , there are a smaller set of callback methods that can be injected into the HTML code, these are:
- (1) my_own_clickmenu1() and my_own_clickmenu1()
- (2) custom_callback1() through custom_callback10()
- (3) cvjs_zoomHere()
If using CADViewer installed through npm, the methods under (1) and (2) above needs to be injected into CADViewer for call-back.
cadviewer.cvjs_setCallbackMethod("custom_callback1", custom_callback1);
//....
cadviewer.cvjs_setCallbackMethod("custom_callback10", custom_callback10);
and declared as methods:
function custom_callback1(){
var id = cadviewer.cvjs_idObjectClicked();
window.alert("Hello callback1 "+id);
};
The injected call-back methods needs to be inserted at the element as the id of that element. Automatically CADViewer will parse the id’s when the modal is opened and return a call to the call-back method if the element is clicked inside the modal.
For plain vanilla JavaScript with class libraries on top-level the application programmer can add any type of functional content to the modal and still be able to capture any interaction through their own code.
Column based content
Inside the Modal, to arrange objects in columns (optionally with an icon), use the CADViewer css class cvjs_modal_1 and arrange each object in a <div>.
my_cvjsPopUpBody = "<div class=\"cvjs_modal_1\" id=\"my_own_clickmenu1()\">Custom<br>Menu 1<br><i class=\'fa fa-undo\'></i></div>";
Row based content
Inside the Modal, to arrange objects in rows, simply use a single <div>, and insert content with a <br> at the end of each line, on a per-line basis.
my_cvjsPopUpBody = "<div>";
my_cvjsPopUpBody += "Element ID: <span style=\"color: darkblue;cursor: pointer;\" id=\"custom_callback1()\" >"+str+"</span><br>";
Sample
Sample code
Below is implemented three sample implementations of the my_cvjsPopUpBody object, which are inserted into the modal at pop-up. Four different menus are randomly displayed.
function myCustomPopUpBody(rmid){
console.log("click on myCustomPopUpBody: "+rmid+" I now change the pop-up menu:");
// make your own popup based on callback
var my_cvjsPopUpBody = "";
// we make 3 random modals
var modalnumber = Math.floor(Math.random() * 6.0);
if (modalnumber == 0){
// standard modal
// standard 3 item menu
// cvjs_modal_1 sets a suitable size
my_cvjsPopUpBody = "<div class=\"cvjs_modal_1\" id=\"my_own_clickmenu1()\">Custom<br>Menu 1<br><i class=\'fa fa-undo\'></i></div>";
my_cvjsPopUpBody += "<div class=\"cvjs_modal_1\" id=\"my_own_clickmenu2()\">Custom<br>Menu 2<br><i class=\'fa fa-info-circle\'></i></div>";
my_cvjsPopUpBody += "<div class=\"cvjs_modal_1\" id=\"cvjs_zoomHere()\">Zoom<br>Here<br><i class=\'fa fa-search-plus\'></i></div>";
}
else
if (modalnumber == 1){
// column based, but just two click menus
my_cvjsPopUpBody = "<div class=\"cvjs_modal_1\" id=\"my_own_clickmenu1()\">Custom<br>Menu One<br><i class=\'fa fa-undo\'></i></div>";
my_cvjsPopUpBody += "<div class=\"cvjs_modal_1\" id=\"cvjs_zoomHere()\">Zoom<br>Here<br><i class=\'fa fa-reply\'></i></div>";
}
else
if (modalnumber >=2){
// row based content with callback
var str = " "+rmid;
my_cvjsPopUpBody = "<div>";
my_cvjsPopUpBody += "Element ID: <span style=\"color: darkblue;cursor: pointer;\" id=\"custom_callback1()\" >"+str+"</span><br>";
var branch = Math.floor(Math.random() * 2.0);
if (branch == 0){
str = " Presumed Wall Void";
my_cvjsPopUpBody += "Survey: <span style=\"color: darkblue;cursor: pointer;\" id=\"custom_callback2()\" >"+str+"</span><br>";
str = " Service Alert";
my_cvjsPopUpBody += "Notice: <span style=\"color: darkblue;cursor: pointer;\" id=\"custom_callback3()\" >"+str+"</span><br>";
}else{
str = "Presumed Ceiling Void";
my_cvjsPopUpBody += "Survey: <span style=\"color: darkblue;cursor: pointer;\" id=\"custom_callback4()\" >"+str+"</span><br>";
str = "Evaluation Pending";
my_cvjsPopUpBody += "Notice: <span style=\"color: darkblue;cursor: pointer;\" id=\"custom_callback5()\" >"+str+"</span><br>";
}
my_cvjsPopUpBody += "Status: <span style=\"color: darkblue;cursor: pointer;\" id=\"custom_callback6()\" >"+"<strong>More Info</strong>"+" <i class=\'fa fa-reply\'></i></span><br>";
my_cvjsPopUpBody += "</div>";
}
return my_cvjsPopUpBody;
}
Modal case 1: - callback for selected items
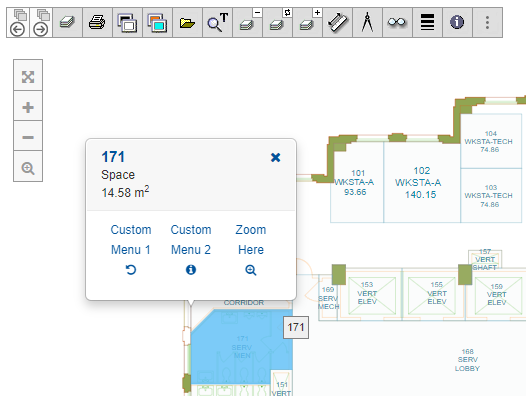
Modal case 2: - callback for selected items
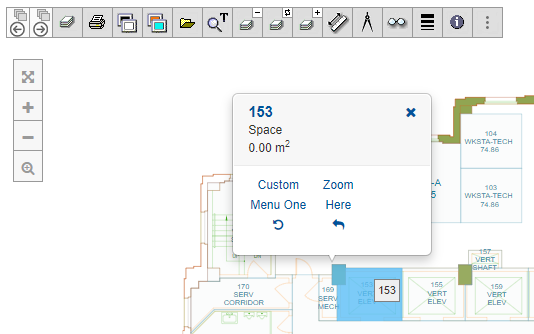
Modal case 3: - callback for selected items
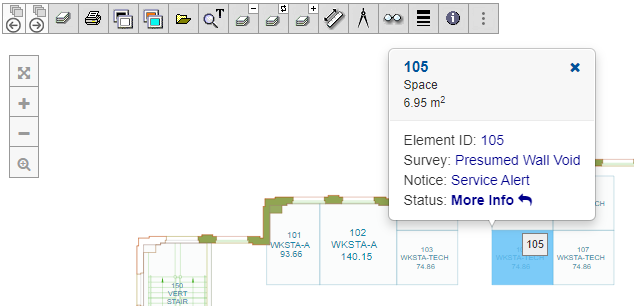
Modal case 4: - callback for selected items
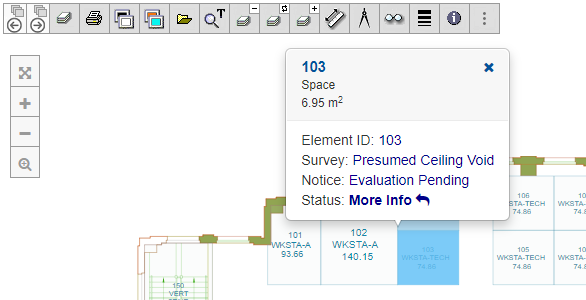
Samples with Custom Modals can be found at our GitHub repositories, cadviewer-script-library and cadviewer-testapp-react-01.
Custom method - populateMyCustomPopUpBody
Typically leave populateMyCustomPopUpBody as is. If a Space Modal is defined with individual <div> then this method can be used to insert values, but typically implementing myCustomPopUpBody gives enough design flexibility.
One way of using the dual methods is to have myCustomPopUpBody contain a stable design with html object id’s defined, and then use populateMyCustomPopUpBody to insert content into locations of those html object ids. In that case, myCustomPopUpBody contains the modal html template that is inserted at time of activation, populateMyCustomPopUpBody does the appropriate data lock up and population of the modal.
function populateMyCustomPopUpBody(rmid, node){
console.log(" we actually have a second callback to change content of the the pop-up menu after myCustomPopUpBody (developed originally for Angular2) populateMyCustomPopUpBody: "+rmid+" "+node);
}
API reference
Click on the method to open the API documentation.
- cvjs_setCallbackForModalDisplay() - optional
- cvjs_InitCADViewer_highLight_popUp_app() - required
Sample implementing dynamic PopUp Modals
Please refere to our Online sample and Sample Documentation for an sample implementing Dynamic PopUp Modals.